Developing Packages for Dynamo for Revit
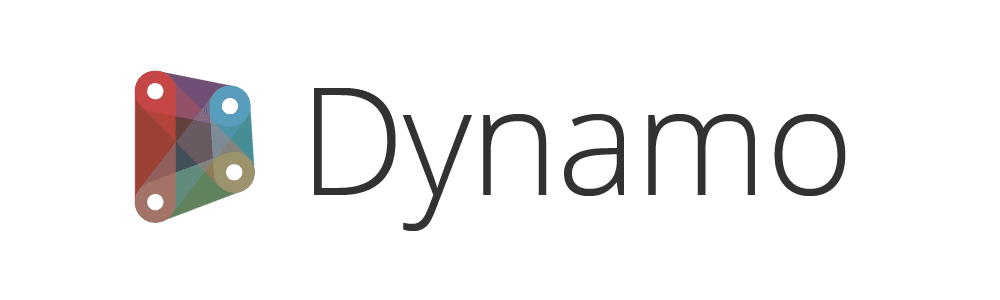
Dynamo for Revit is a powerful visual programming extension for Autodesk Revit, allowing users to automate repetitive tasks and create custom workflows. Developing packages for Dynamo requires an understanding of both the .NET framework and the specifics of Revit’s API. This article guides you through creating a Dynamo package targeting both .NET Framework 4.8 and .NET 8, incorporating Windows Forms and WPF without targeting .NET Standard 2.0.
Prerequisites
Before diving into package development, ensure you have the following:
- Visual Studio 2022 (or later)
- Revit 2023 or later (with Dynamo)
- .NET Framework 4.8 and .NET 8 SDK
- Dynamo Revit API Docs and Revit API Docs
Setting Up Your Development Environment
- Install Required SDKs and Tools:
- Download and install .NET 8 SDK from Microsoft.
- Install Visual Studio 2022 with .NET desktop development.
- Configure Your Project:
- Open Visual Studio and create a new solution.
- Add a Class Library project to the solution that targets both .NET Framework 4.8 and .NET 8.
Creating a Multi-Target Framework Project
You can simplify your project setup by including both target frameworks in a single project. This ensures you write the code once and maintain a consistent approach. Here’s how to configure your project file (.csproj
) for multi-targeting:
- Edit Your Project File:
- Right-click on the project in Solution Explorer and choose "Edit Project File."
- Add the following configurations:
1<Project Sdk="Microsoft.NET.Sdk.WindowsDesktop">
2
3<PropertyGroup>
4<OutputType>Library</OutputType>
5<TargetFrameworks>net48;net8.0-windows</TargetFrameworks>
6<UseWPF>true</UseWPF>
7<UseWindowsForms>true</UseWindowsForms>
8</PropertyGroup>
9
10<PropertyGroup Condition="'$(Configuration)|$(Platform)' == 'Debug|AnyCPU'">
11<DefineConstants>DEBUG;TRACE</DefineConstants>
12<DebugType>portable</DebugType>
13<PlatformTarget>AnyCPU</PlatformTarget>
14<ErrorReport>prompt</ErrorReport>
15<CodeAnalysisRuleSet>MinimumRecommendedRules.ruleset</CodeAnalysisRuleSet>
16</PropertyGroup>
17
18<ItemGroup Condition="'$(TargetFramework)' == 'net48'">
19<Reference Include="RevitAPI">
20<HintPath>path\to\RevitAPI.dll</HintPath>
21</Reference>
22<Reference Include="RevitAPIUI">
23<HintPath>path\to\RevitAPIUI.dll</HintPath>
24</Reference>
25</ItemGroup>
26
27<ItemGroup Condition="'$(TargetFramework)' == 'net8.0-windows'">
28<PackageReference Include="RevitAPI" Version="2023.0.0" />
29<PackageReference Include="RevitAPIUI" Version="2023.0.0" />
30</ItemGroup>
31
32</Project>
Implementing Windows Forms/WPF
- Adding Forms and Windows:
- Right-click on the project, select "Add" -> "New Item," and choose "Windows Form" or "WPF Window."
- Design your form or window using the designer tools provided by Visual Studio.
- Dynamo Integration:
- Implement a basic Dynamo NodeModel. Here’s an example of a simple node that opens a Windows Form:
1using Dynamo.Wpf;
2using Dynamo.Graph.Nodes;
3using System.Windows.Forms;
4
5[NodeName("Show Form")]
6[NodeCategory("Custom Nodes")]
7[NodeDescription("Opens a Windows Form")]
8public class ShowFormNode : NodeModel
9{
10 public ShowFormNode()
11 {
12 InPorts.Add(new PortModel(PortType.Input, this, new PortData("input", "Input data")));
13 OutPorts.Add(new PortModel(PortType.Output, this, new PortData("output", "Output data")));
14 RegisterAllPorts();
15 }
16
17 protected override void OnEvaluate()
18 {
19 Form form = new Form();
20 form.ShowDialog();
21 // Dummy output
22 this.Value = "Form Shown";
23 }
24}
Building and Packaging Your Dynamo Nodes
- Build the Project:
- Ensure the project builds successfully for both target frameworks. Address any compatibility issues that arise, particularly with references and API differences.
- Create the Dynamo Package:
- Use Dynamo's package manager to create a new package.
- Include the DLLs for both target frameworks in the package, ensuring they are properly referenced in the
pkg.json
file. - Here’s an example
pkg.json
structure:
1{
2 "name": "CustomNodes",
3 "version": "1.0.0",
4 "description": "Custom Dynamo nodes for Revit.",
5 "keywords": ["Revit", "Dynamo"],
6 "dependencies": {
7 ".NETFramework,Version=v4.8": "path/to/net48/dll",
8 ".NETCoreApp,Version=v8.0": "path/to/net8/dll"
9 },
10 "node_libraries": ["CustomNodes, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null"]
11}
Testing Your Package:
- Install the package in Dynamo and test each node.
Ensure the nodes work seamlessly in both environments, and the forms/windows appear as expected.
Conclusion
Developing packages for Dynamo for Revit targeting both .NET Framework 4.8 and .NET 8 allows you to leverage the strengths of each framework. By configuring your project to target both frameworks, you can write your code once and maintain a consistent approach. This ensures compatibility with a wide range of Revit versions and enables your nodes to utilize modern .NET capabilities. By following this guide, you can create robust and versatile Dynamo nodes that enhance Revit workflows.
Happy coding!